- Published on
Stock Price Prediction with Apache Spark and Apache Cassandra.
- Authors
- Name
- Victor Oketch Sabare
- @sabare12
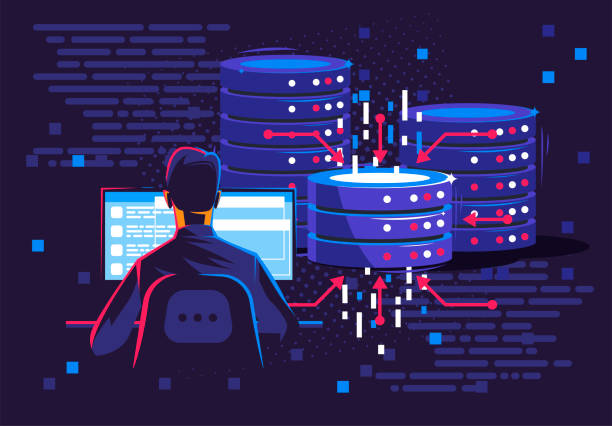
Introduction
Stock price prediction is a vital part of financial analysis, and it can be a daunting task to obtain and process data to make accurate predictions. Apache Spark and Apache Cassandra can be powerful tools in building a data pipeline for stock price prediction. In this article, we will explore the process of building a data pipeline for stock price prediction using Apache Spark and Apache Cassandra.
Apache Spark is a distributed computing system that provides efficient data processing capabilities. Apache Cassandra is a NoSQL database that can handle large amounts of data in a distributed environment. A data pipeline is essential for stock price prediction as it involves obtaining, processing, and analyzing data to make accurate predictions.
Data Collection
There are different data sources for obtaining stock data, such as Yahoo Finance, Google Finance, and Alpha Vantage. Alpha Vantage is a financial data provider that offers free and paid APIs for obtaining stock data. In this article, we will use the Alpha Vantage API to obtain stock data.
The following Python code shows how to obtain stock data from the Alpha Vantage API and store it in Apache Cassandra:
from alpha_vantage.timeseries import TimeSeries
from cassandra.cluster import Cluster
ts = TimeSeries(key='YOUR_API_KEY', output_format='pandas')
symbol = 'AAPL'
data, meta_data = ts.get_daily_adjusted(symbol=symbol, outputsize='full')
cluster = Cluster(['127.0.0.1'])
session = cluster.connect('stock_prices')
for index, row in data.iterrows():
session.execute(
"""
INSERT INTO stock_data (symbol, date, open, high, low, close, adjusted_close, volume)
VALUES (%s, %s, %s, %s, %s, %s, %s, %s)
""",
(symbol, str(index), row['1. open'], row['2. high'], row['3. low'], row['4. close'], row['5. adjusted close'], row['6. volume'])
)
Data Preprocessing
Before building a model for stock price prediction, it is essential to preprocess the data to make it ready for analysis. The following steps can be taken to preprocess the data:
Cleaning the data: Remove any duplicates, incorrect data, or data that is not relevant to the analysis.
Handling missing values: Fill in any missing values using techniques such as interpolation or imputation.
Data normalization: Scale the data to a standard range to make it comparable across different variables.
Feature Engineering
Feature engineering involves creating new features from the existing data to make it more informative. In stock price prediction, the following features can be engineered:
Calculation of technical indicators: Technical indicators such as moving averages, relative strength index (RSI), and moving average convergence divergence (MACD) can provide valuable insights into the stock's performance.
Adding sentiment analysis: Analyzing news articles or social media posts related to the stock can provide valuable insights into investor sentiment.
Adding macroeconomic indicators: Macroeconomic indicators such as GDP, inflation, and interest rates can impact the stock market and provide valuable insights into the stock's performance.
Model Building
After preprocessing the data and engineering features, we can build a machine learning model to predict the stock price. The following steps can be taken to build a model:
Choosing machine learning algorithms: There are various machine learning algorithms that can be used for stock price prediction, such as linear regression, decision trees, and random forests.
Splitting data into training and testing sets: Split the data into a training set to train the model and a testing set to evaluate the model's performance.
Model training and evaluation: Train the model using the training set and evaluate its performance using the testing set. We can use evaluation metrics such as mean squared error (MSE)
The following Python code shows how to split the data into training and testing sets and train the model using linear regression:
from sklearn.linear_model import LinearRegression
from sklearn.model_selection import train_test_split
from sklearn.metrics import mean_squared_error
# Split the data into training and testing sets
X = data.drop(['symbol', 'date', 'close'], axis=1)
y = data['close']
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
# Train the model using linear regression
lr = LinearRegression()
lr.fit(X_train, y_train)
# Evaluate the model using mean squared error
y_pred = lr.predict(X_test)
mse = mean_squared_error(y_test, y_pred)
print("Mean squared error:", mse)
Integration with Apache Spark
Apache Spark can be integrated with Apache Cassandra to perform data analysis and predictions. The following steps can be taken to integrate Apache Spark and Apache Cassandra:
Install the Spark-Cassandra connector: The connector allows Spark to read and write data from Apache Cassandra.
Create a Spark session: The Spark session can be used to interact with Spark.
Read data from Apache Cassandra: Read the data from Apache Cassandra using Spark.
Perform data analysis and predictions: Perform data analysis and predictions using Spark.
Conclusion
In this article, we have explored the process of building a data pipeline for stock price prediction using Apache Spark and Apache Cassandra. We have discussed the importance of data pipeline for stock price prediction, data collection, data preprocessing, feature engineering, model building, and integration with Apache Spark. Future work can involve exploring other machine learning algorithms and techniques to improve the accuracy of stock price prediction.
Link to the GitHub repository containing the full code for this project: https://github.com/sabareh/stock-price-prediction-spark-cassandra